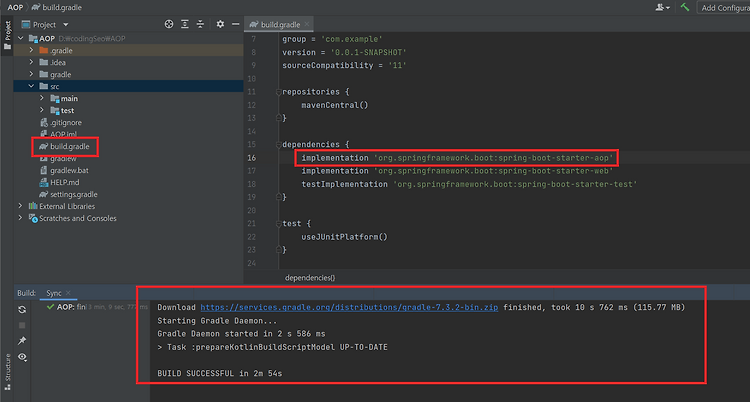
[Spring] AOP란?
@욕심쟁이
·2022. 1. 22. 19:10
반응형
AOP 프로젝트 세팅
gradle을 통해 다운
dependencies에
implementation 'org.springframework.boot:spring-boot-starter-aop' // 추가
controller RestApiController 생성
package com.example.aop.controller;
import com.example.aop.dto.User;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class RestApiController {
@GetMapping("/get/{id}")
void get(@PathVariable Long id, @RequestParam String name){
System.out.println("id = " + id + ", name = " + name);
}
@PostMapping("/post")
void post(@RequestBody User user){
System.out.println("post method " + user);
}
}
DTO User.class 생성
package com.example.aop.dto;
public class User {
private String id;
private String pw;
private String email;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPw() {
return pw;
}
public void setPw(String pw) {
this.pw = pw;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "User{" +
"id='" + id + '\'' +
", pw='" + pw + '\'' +
", email='" + email + '\'' +
'}';
}
}
AOP 를 만들어 설정
package com.example.aop.aop;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;
import java.lang.reflect.Method;
@Aspect //흩어진 관심사를 모듈화 한 것. 주로 부가기능을 모듈화함.
@Component
public class ParameterAop {
@Pointcut("execution(* com.example.aop.controller..*.*(..))") //내가 어느부분에 적용시킬것인지 => 특정 controller하위
private void pointcut(){
}
@Before("pointcut()") //메서드 실행전(언제 실행할것이냐) => pointcut실행되는 지점
public void before(JoinPoint joinPoint){ //joinPoint 라는 객체 를 가지고 있음
MethodSignature methodSignature = (MethodSignature) joinPoint.getSignature();
Method method = methodSignature.getMethod();
System.out.println(method.getName()); // 어떤메서드를 실행했는지 확인
Object[] arg = joinPoint.getArgs();
for (Object obj : arg){
System.out.println("type = " + obj.getClass().getSimpleName());
System.out.println("value = " + obj);
}
}
@AfterReturning(value = "pointcut()", returning = "returnodj") //메서드 실행이 되어을때 반환값이 알고싶을때 => pointcut실행되는 지점
public void afterReture(JoinPoint joinPoint, Object returnodj){ // object값을 가짐
System.out.println("joinPoint = " + joinPoint + ", returnodj = " + returnodj);
}
}
post
get
반응형
'IT > Spring' 카테고리의 다른 글
[Spring] 주요 Annotation (0) | 2022.01.19 |
---|---|
[Springboot] RESTful GET 해보기! (0) | 2022.01.18 |
[Springboot] RESTful POST 해보기! (0) | 2021.11.06 |
[Springboot] 프로젝트 생성하기 (0) | 2021.11.05 |